Working with .NET in Dynamics NAV 2018 is cool. It feels like you are writing C/AL armed with the full might and strength of an Asgardian god. There is a good amount of tutorials online that help us understand how this interoperability works.
Recently, I was in need to model a List<T> where T could be any type of class. I will demonstrate how this can be achieved. As an example, we will attempt to model a NAV Purchase Order and NAV Purchase Lines.
Step 1: Create a .NET Standard Class Library in Visual Studio
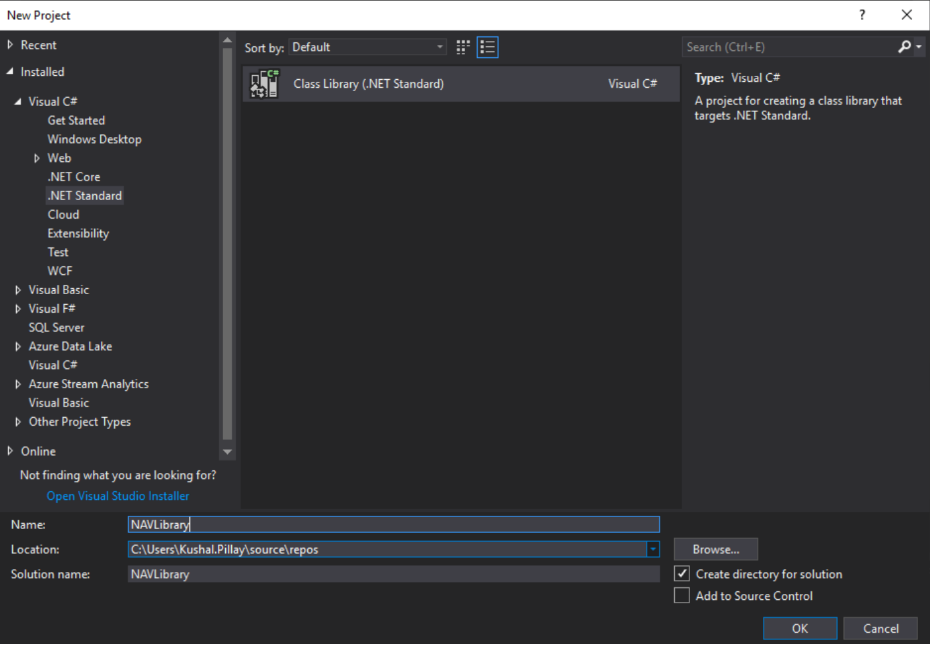
Step Two: The Purchase Order Class and Purchase Lines Class
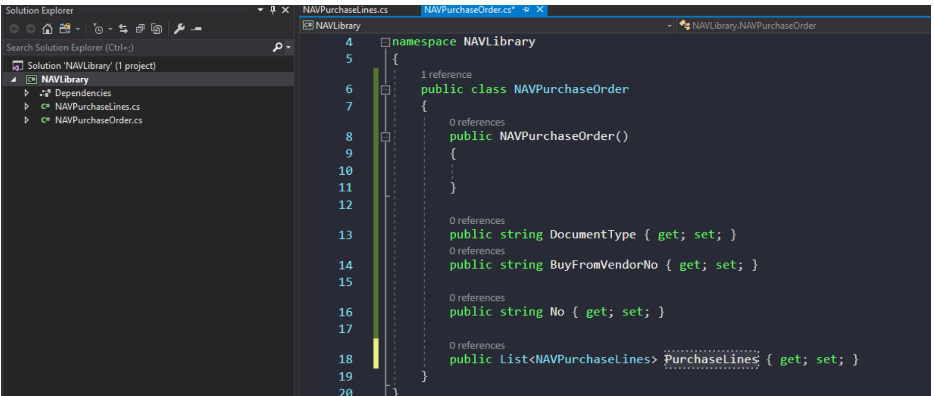
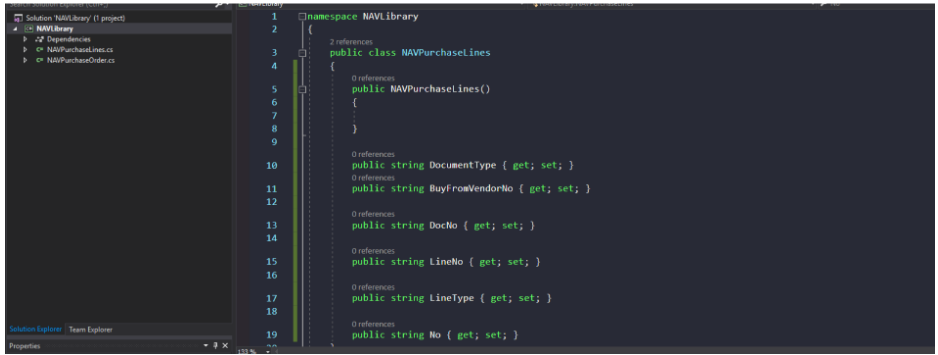
Step Three: The C/AL Code
PurchaseOrder := PurchaseOrder.NAVPurchasOrder();
PurchaseOrder.DocumentType := ‘Order’;
PurchaseOrder.No := ‘PO1000’;
Now its time to set values for our Items. But how do we do that? Lets say there are 2 Items in this PO. We would first have to
REPEAT
//Somehow add NAV Items to a List<NAVItems>
UNTIL NAVPurchaseLine.NEXT = 0
First, we need a List Variable:
NavItemsList: System.Collections.Generic.List`1.’mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089'
Next, we need to Tell NAV that this List is of type dotnet NAVPurchaseLines. To do this, I figured out a quick and easy way using the NavItemsList constructor. C/AL will make this of Type whatever the constructor returns.
So in our Purchase Order class, we can create a function that returns a List<NAVPurchaseLines> as such:
public List<NAVPurchaseLines> InitializeNavPurchaseLines()
{
return new List<NAVPurchaseLines>();
}
in C/AL:
NavItemsList := PurchaseOrder. InitializeNavPurchaseLines();
Now we can loop through the Items:
NavItem: .NET Type of NAVLibrary.PurchaseLines
REPEAT
NavItem:=NavItem.NAVItem;
NavItem.DocumentType:= ‘Order’;
NavItem.BuyFromVendorNo:= ‘31314’;
NavItem.DocNo:= ‘PO1000’;
NavItem.LineNo:= ‘1000’;
NavItemsList.Add(NavItem);
UNTIL NAVPurchaseLine.NEXT = 0;
And Finally, we can add our NavItemsList to our Purchase Order class
Type := GETDOTNETTYPE(PurchaseOrder);
Type.GetProperty(‘PurchaseLines’).SetValue(PurchaseOrder,NavItemsList);
Which this technique, We are easily able to add Any Type of List to our Class from C/AL!
SO.MUCH.POWER
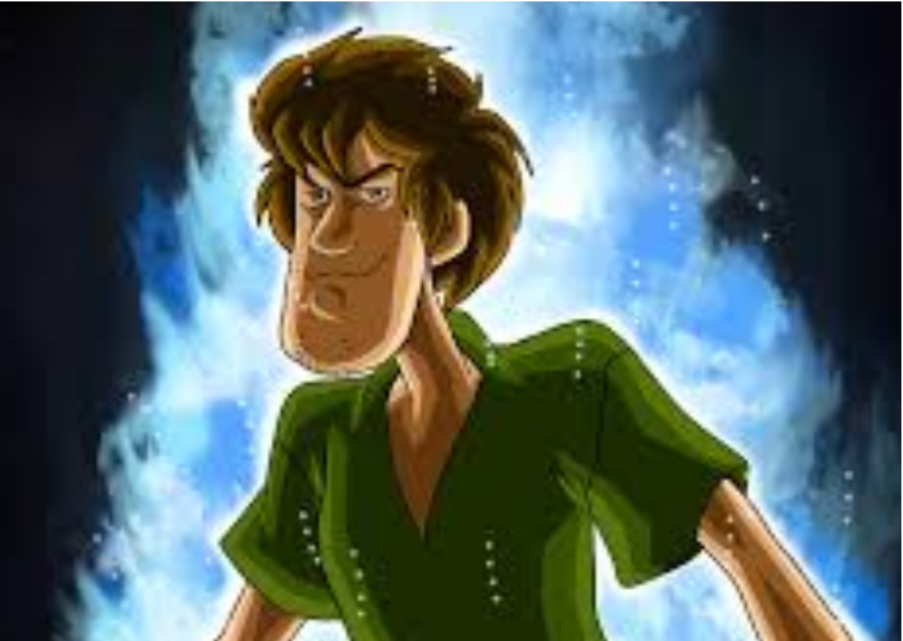